Django on the Go: Harnessing the Power of Django in Termux on Android
Django on the Go: Harnessing the Power of Django in Termux on Android
Couldn't load pickup availability
Book overview
This outline covers the basics of Django, its integration with Android through Termux, and practical projects and advice for real-world applications. It's designed to be comprehensive for developers at various levels of expertise.
Django on the Go: Harnessing the Power of Django in Termux on Android is an innovative guide for developers who are eager to explore the fusion of Django’s robust web development capabilities with the flexibility of the Android platform through Termux. This book is a treasure trove of knowledge, offering a unique perspective on leveraging a mobile device to develop, test, and deploy powerful web applications using Django. From setting up your Android device with Termux to deploying fully-fledged web applications, this book covers it all with practical examples, expert insights, and step-by-step instructions.
Who This Book is For
Web developers seeking to expand their skills into mobile-based development.
Django enthusiasts curious about leveraging Termux on Android for web application projects.
Students, educators, and hobbyists interested in exploring unconventional yet powerful development environments.
Key Topics Covered
Introduction to Django and Termux: Understand the fundamentals of Django and how Termux transforms your Android device into a Linux-based development environment.
Environment Setup: Step-by-step guidance on setting up Django within Termux, including Python installation, database configuration, and essential packages.
Developing with Django: Dive deep into Django’s models, views, templates, and ORM system with practical examples and real-world scenarios.
Mobile-Optimized Development: Tips and tricks for efficient coding on a mobile device, dealing with limitations, and leveraging mobile-specific features.
Advanced Django Features: Explore asynchronous task handling, RESTful API development, and integrating front-end technologies.
Security and Performance: Best practices for securing your Django applications and optimizing performance within the Termux environment.
Real-World Projects: Comprehensive projects that guide you through building and deploying a Django application from scratch on your Android device.
Introduction
Welcome to Django on the Go: Harnessing the Power of Django in Termux on Android, a pioneering book that opens doors to a new world of web development possibilities. In this book, you will journey through the exciting intersection of Django’s powerful web framework and the versatility of the Android platform via Termux. Whether you’re developing from a café, in transit, or from the comfort of your couch, this book empowers you to build impressive web applications directly from your Android device. Get ready to transform your mobile device into a powerful development workstation as you embark on this unique and thrilling learning adventure!
This book is designed to be an engaging, comprehensive guide for anyone looking to combine the power of Django with the convenience of mobile development using Termux on Android. The aim is to provide readers with the knowledge and tools they need to harness this unique development environment effectively.
Table Of Contents
Overview of Django Framework 13
Introduction to Termux: A Linux Terminal Emulator for Android 14
Setting up the Development Environment 16
Installing Python and Django on Android in Termux 16
Update and Upgrade Packages 16
Run the Django Development Server 18
Access Development Server from Other Devices (Optional) 20
Configuring Termux for Django Development 21
Update and Upgrade Packages 21
Configure Virtual Environments 27
Set Up Storage and Permissions 33
Configure Networking (Optional) 33
Using Text Editors and IDEs 33
Explore Additional Packages 34
Essential Tools and Packages 34
Text Editors (Vim, Nano, Emacs) 36
Database Systems (SQLite, PostgreSQL, MySQL) 42
Python Packages (Requests, Flask, etc.) 42
Django Project and App Structure 44
Creating a Project and an App 45
Differences projects and apps 46
Understanding Models, Views, and Templates 48
Developing a Simple Django Application 52
Creating a Basic Blog Application 52
Step 1: Set Up Your Django Project 52
Step 7: Run the Development Server 56
Integrating with SQLite Database 56
Advantages of Using SQLite with Django 59
Implementing CRUD Operations 60
Step 2: Create and Apply Migrations 60
Step 3: Create Views for CRUD Operations 61
Step 7: Run the Development Server 64
Termux editors to edit code from Django 64
Code Editor (Via Termux API) 66
User Authentication and Authorization 67
Extending the Authentication System 68
Token-Based Authentication: 69
Working with Django REST Framework 69
Using ViewSets and Routers: 71
Authentication & Permissions 72
Asynchronous Tasks with Celery 73
Setting Up Celery with Django 73
Deploying Django Apps on Servers 76
Regular Updates and Backups 78
Tips for Efficient Deployment on Termux Android Devices 79
Case Studies of Django in Android Termux 85
Advanced Project Ideas and Implementation 88
Learning Management System (LMS) 88
Content Management System (CMS) 89
Healthcare Management System 89
Real Estate Listing Platform 89
Event Management and Ticketing System 90
General Implementation Tips 91
Troubleshooting and Best Practices 91
Common Issues in Django and Termux Android Use 92
Dependency Installation Issues 92
Best Practices for Django Development in Termux 93
Keep Your Environment Updated 93
Test on a Standard Development Environment 93
Optimize for Mobile Development 93
Performance Optimization Techniques 94
Use of Content Delivery Network (CDN) 95
Efficient Use of Middleware 95
Database Connection Pooling 95
Code Profiling and Monitoring 95
Upgrade to Latest Django Version 96
Scalable Hosting Environment 96
Best Practices in Django Development 97
Use Django's Built-in Features 97
Write Readable and Consistent Code 98
Responsive and Mobile-Friendly Design 99
Error Logging and Monitoring 99
Future Trends and Resources 100
Upcoming Features in Django and Android Termux Development 100
Future Trends in Django Development 100
Upcoming Features in Android Termux Development 101
Resources for Staying Updated 101
Additional Resources and Communities 102
Common Communities and Platforms 103
Django in Android and Termux 104
Development and Deployment 104
Best Practices and Troubleshooting 105
Future Trends and Resources 106
Final Thoughts and Advice for Continuous Learning 106
Embrace a Learning Mindset 106
Balance Theory and Practice 107
Document and Share Your Knowledge 107
Prioritize Quality and Best Practices 107
Continuous Learning Resources 107
Contribute to the Community 108
Overview of Django Framework
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It's known for its simplicity and robustness, making it a popular choice for web developers. Here's an overview of its key features:
MTV Architecture: Django follows the Model-Template-View (MTV) architectural pattern, similar to the MVC (Model-View-Controller) architecture. This structure separates data handling (Model), user interface (Template), and business logic (View), promoting organized coding and easy maintenance.
ORM (Object-Relational Mapping): Django's ORM allows developers to interact with the database using Python code instead of SQL. This abstraction simplifies database operations and ensures database-agnostic code.
Admin Interface: Django comes with a built-in, ready-to-use admin interface that lets you manage data. It's a powerful feature for handling database operations through a user-friendly web interface.
Development Speed: Django aims to make development faster and easier with a "batteries-included" approach, providing built-in features for common web development tasks.
Security: Django emphasizes security and helps developers avoid common security mistakes, such as SQL injection, cross-site scripting, cross-site request forgery, and clickjacking. Its user authentication system provides a secure way to manage user accounts and passwords.
Scalability: Django is designed to be scalable, making it suitable for handling high traffic and large-scale projects.
Versatility: It's used for all types of web applications, from content management systems and wikis to social networks and news sites.
Vibrant Community: Django has a strong and active community, providing a wealth of resources, third-party packages, and support.
Documentation: Django is well-documented, making it accessible for beginners and providing detailed guidance for all levels of development.
Django's design philosophies include DRY (Don't Repeat Yourself) and "explicit is better than implicit", encouraging clean and readable code. Its popularity and wide adoption can be attributed to these principles and features, making it a top choice for modern web development.
Introduction to Termux: A Linux Terminal Emulator for Android
Termux is an Android application that offers a powerful and versatile Linux terminal emulator. It's an open-source app that provides a command-line environment and a collection of Linux packages on Android devices. Here's a brief introduction to Termux:
Key Features of Termux
Linux Environment
Termux turns your Android device into a lightweight Linux environment. It does not require rooting your device and works on most Android versions.
Package Management
It comes with a built-in package manager (apt) that allows you to install a wide range of Linux packages. This includes popular tools like Python, Git, Node.js, and more.
Programming
Termux supports various programming languages, including Python, PHP, Ruby, and Java. It's a great tool for developers who want to write and test code directly on their mobile devices.
SSH and Remote Access
You can use Termux for SSH access to remote servers, making it useful for managing servers and cloud services directly from your Android device.
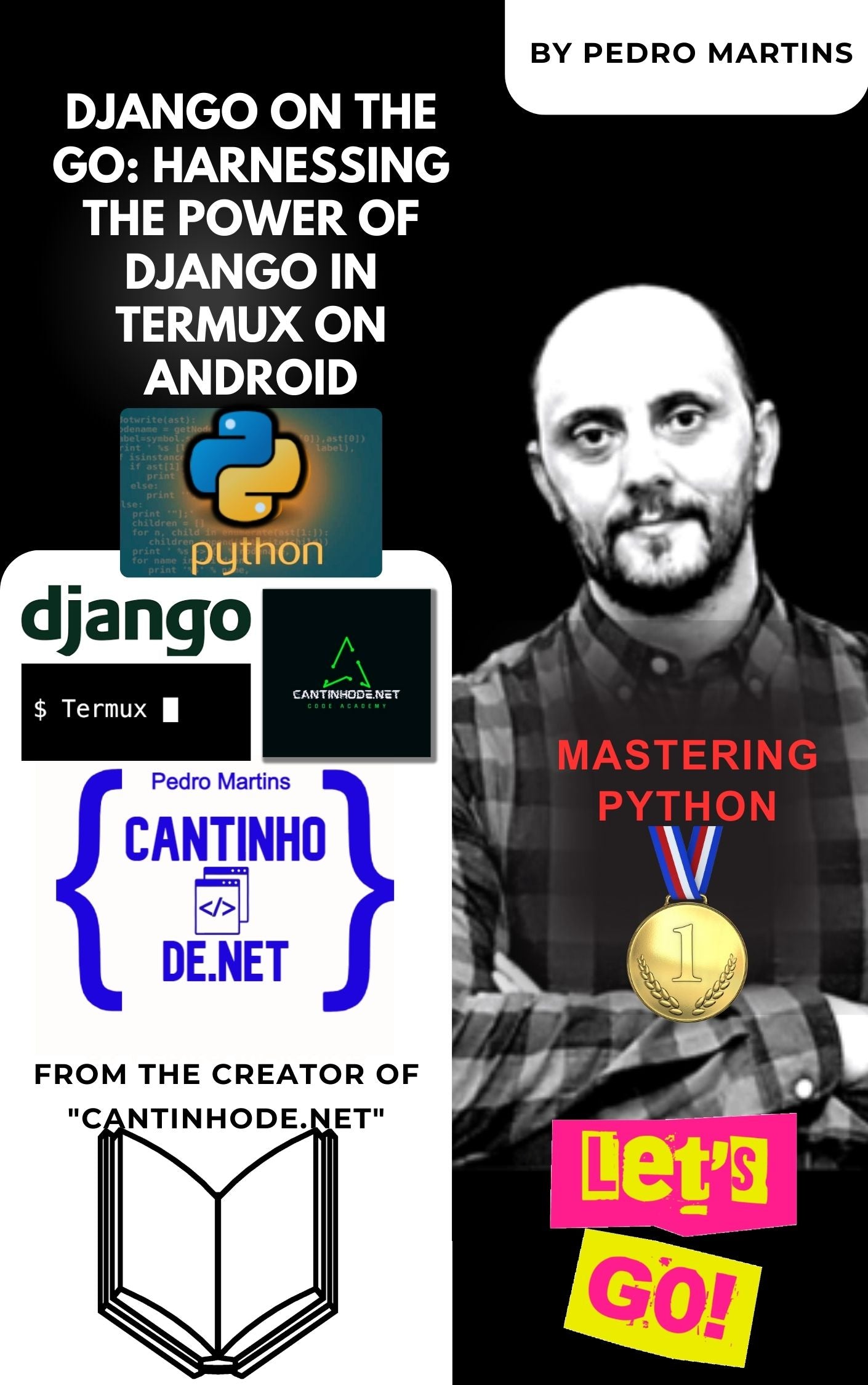